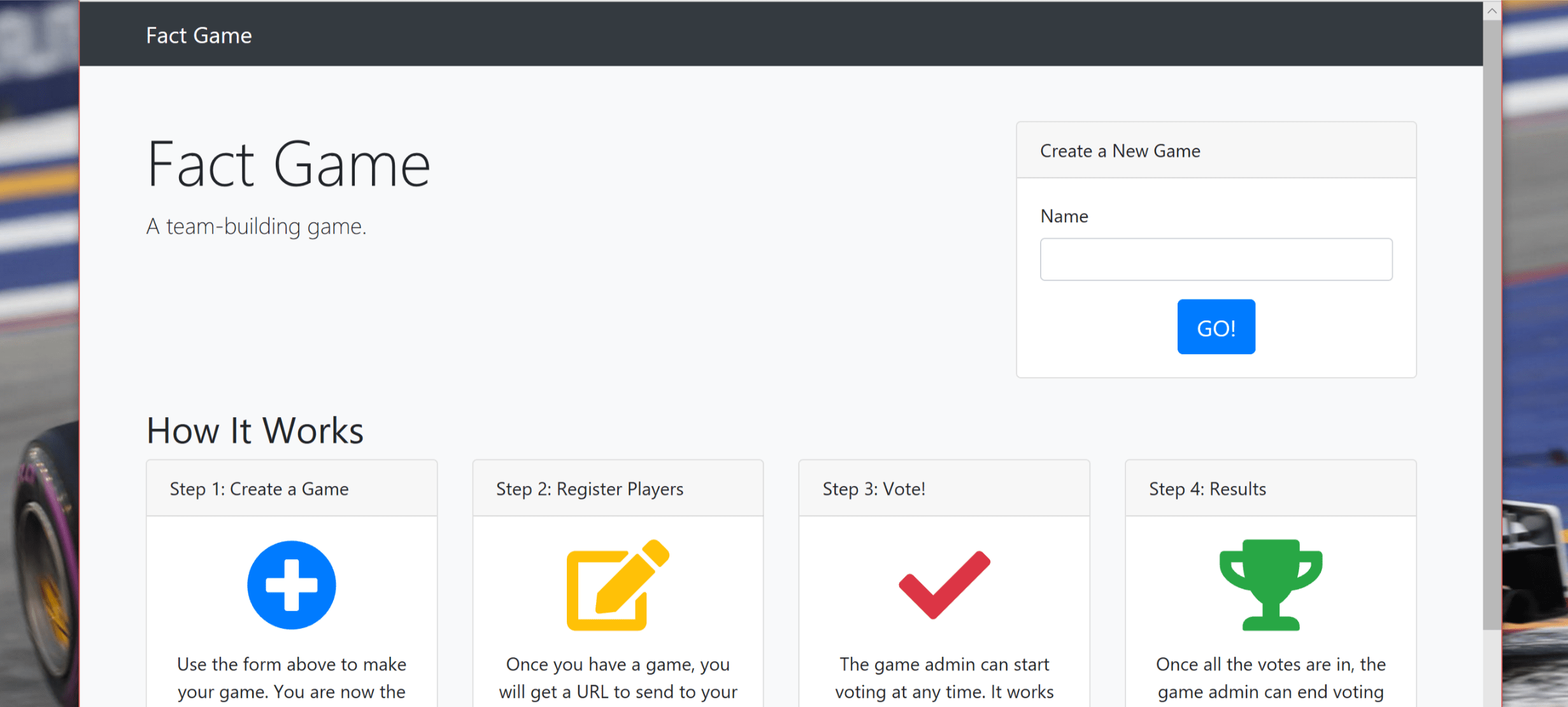
Fact Game
Making a simple game in ASP.NET Core.
I work at AWH. Once a year, we have a day long company meeting. We get updates on how the company did last year and what we are planning for the coming year. We also play some games. Some involve cards, some involve starships. Then there is the fact game.
The Game
The concept is simple: every person playing writes down an interesting fact about themselves that nobody else knows. We then read those facts out to the group, write them on a board, then everyone votes on who they think wrote each fact. Each fact is worth the same numbers of points as there are players. Anyone who guesses right gets the points split with anyone else who also guessed right. If no one guesses a fact, the person who wrote it gets all the points.
The Problems
Each year, we run into some issues.
- Drawing the board. We use big dry-erase board and make a grid. Facts go down the left side and names along the top. Drawing this grid each year is annoying.
- Crowding. Each player chooses a symbol (and color) and draws it by their name at the top. Voting happens by placing their symbol in the various boxes. There are usually lots of us trying to vote at a time so we get in each other鈥檚 way quite a bit.
- Scoring. Not really hard, but someone has to actually do it.
- Coming up with the fact. It get鈥檚 harder each year. This year, we had no advanced notice to come up with our fact which made it even more difficult.
These are really annoyances rather than problems, but they still got us thinking about how we could develop something to solve some of these problems. We are developers after all, and we like to solve problems.
My Solution: Fact Game
So I made a game and here it is.
Play is basically the same as the original. One person has to be the game admin and create the game. They are then given some URLs: one for the players, and one for accessing the admin page. The game has three stages: registering, voting, and closed.
When the game is registering, players can use their URL to sign up. They provide their name, their fact, a symbol (by picking a Font Awesome icon), and a color. They can update this information freely until voting beings. The admin page during this stage shows the list of players registered and a button to start voting. Registration for players is stored in browser cookies, so there are no passwords to create or remember. You can even be a player in multiple games at a time.
Once the admin has triggered voting, the player鈥檚 URL (which doesn鈥檛 change between stages) becomes their ballot. Each fact is presented to them with a dropdown list of all the players. They can update their votes freely until the game is closed. The admin page shows the grid during this stage. Like the dry-erase board, it shows the players across the top (with their symbol) and facts down the left. Any submitted votes are shown in the grid using the symbol and color the player chose.
Once the admin closes the game, every player鈥檚 ballot is scored according to the rules above, and they can see their results using the player URL. The admin URL in this stage still shows the grid, but now highlights the correct squares and shows a leaderboard with all the player鈥檚 and their scores.
Development
The site is my first dip into .NET Core, ASP.NET Core, and ASP.NET MVC Core. My first impressions are positive. I used Visual Studio instead of the CLI. From what I can tell, there is very little difference in the resulting project, but Visual Studio holds your hand a bit more.
Controllers are basically the same as I鈥檓 used to working in previous MVC versions. Views are very different, though, and I like them a lot. Tag Helpers rubbed me the wrong way at first, but I鈥檝e come around on them and building the views was very enjoyable. Configuration was kind of annoying to figure out, but I eventually got it working. Documentation around everything is still a work-in-progress, so expect some gaps every now and then.
The site is hosted on a Azure using a free App Service plan. I love using Azure, and while the free tier is a bit slow (and goes to sleep often), it鈥檚 still very nice to use and, most importantly, free. Deployments from Visual Studio to Azure are also a breeze.
Data storage was a challenge. I initially build it with an embedded SQLite database, which worked fine but came with a big drawback: no concurrency. More than one user at a time could bring it down. Finding a hosted database was harder than expected as I didn鈥檛 want to pay anything. I eventually settled on using MongoDB and mLab (formerly MongoLab) due to the free tier which only has a storage limit of 500MB. If this somehow takes off, I might transition to Azure Table Storage as it鈥檚 similar enough to MongoDB that it would not require a lot of reworking and is a lot cheaper than mLab. If Azure Table Storage had a free tier, I鈥檇 already be using it.
Future Improvements
One of my favorite things about this little project is that I used no custom CSS or JavaScript. All the styles are handled by Bootstrap 4 and all the behavior is basic HTML forms and server-side logic.
That being said, there is a place for some custom front-end code: the reveal. At the end of the game, we go down the board and reveal who each fact belongs to and find out who got that one right. The new game has no reveal. Once voting is closed, all the answers are shown immediately and at once. I imagine the admin page would be shown on the projector during and after voting, so having a reveal that everyone could watch would be nice.
I also think I might add a way to move backwards through the stages. Currently the admin can move the game from registering to voting, but not the other way around. I think this could be helpful in many circumstances. It鈥檚 not a challenging thing to implement, I just didn鈥檛 bother with the first pass.
Then there is the text throughout. I think I did an alright job of explaining the game on the home page and with bit of text around the other pages, but it could probably be better in places. I鈥檓 also missing any sort of meta tags for SEO and could use a logo.
Oh, and the code could use a lot of refactoring. It鈥檚 messy, to say the least.